wavesurfer.js
New TypeScript version
wavesurfer.js v7 beta is a TypeScript rewrite of wavesurfer.js that brings several improvements:
- Typed API for better development experience
- Enhanced decoding and rendering performance
- New and improved plugins
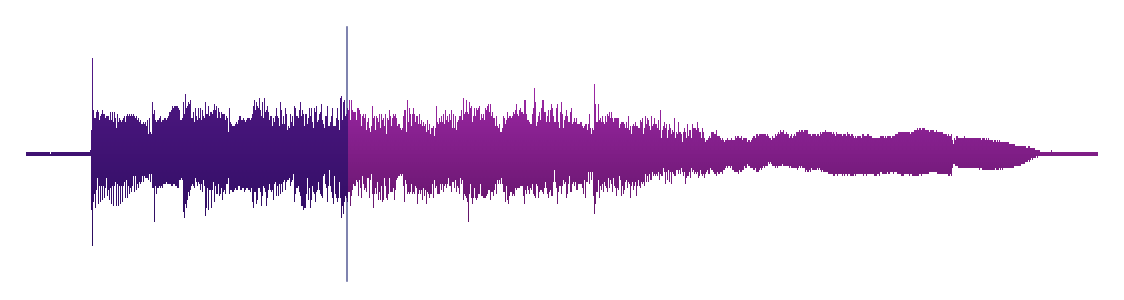
Try it out:
npm install --save wavesurfer.js@beta
import WaveSurfer from 'wavesurfer.js'
Alternatively, import it from a CDN as a ES6 module directly in the browser:
<script type="module">
import WaveSurfer from 'https://unpkg.com/wavesurfer.js@beta'
const wavesurfer = WaveSurfer.create({ ... })
</script>
Or, as a UMD script tag which exports the library as a global WaveSurfer
variable:
<script src="https://unpkg.com/wavesurfer.js@beta/dist/wavesurfer.min.js"></script>
To import a plugin, e.g. the Timeline plugin:
import Timeline from 'https://unpkg.com/wavesurfer.js@beta/dist/plugins/timeline.js'
TypeScript types are included in the package, so there's no need to install @types/wavesurfer.js
.
See more examples.
Documentation
See the documentation on wavesurfer.js methods, options and events on our website.
Plugins
The "official" plugins have been completely rewritten and enhanced:
- Regions โ visual overlays and markers for regions of audio
- Timeline โ displays notches and time labels below the waveform
- Minimap โ a small waveform that serves as a scrollbar for the main waveform
- Envelope โ a graphical interface to add fade-in and -out effects and control volume
- Record โ records audio from the microphone and renders a waveform
- Spectrogram โ visualization of an audio frequency spectrum
CSS styling
wavesurfer.js v7 is rendered into a Shadow DOM tree. This isolates its CSS from the rest of the web page.
However, it's still possible to style various wavesurfer.js elements via CSS using the ::part()
pseudo-selector.
For example:
#waveform ::part(cursor):before {
content: '๐';
}
#waveform ::part(region) {
font-family: fantasy;
}
You can see which elements you can style in the DOM inspector โ they will have a part
attribute.
See this example for play around with styling.
Upgrading from v6
Most options, events, and methods are similar to those in previous versions.
Notable differences
- The
backend
option is removed โ HTML5 audio (or video) is the only playback mechanism. However, you can still connect wavesurfer to Web Audio viaMediaElementSourceNode
. See this example. - The Markers plugin is removed โ use the Regions plugin with just a
startTime
. - No Microphone plugn โ superseded by the new Record plugin with more features.
- No Cursor and Playhead plugins yet โ to be done.
Removed options
backend
,audioContext
,closeAudioContext', 'audioScriptProcessor
โ there's no Web Audio backend, so no AudioContextautoCenterImmediately
โautoCenter
is now always immediate unless the audio is playingbackgroundColor
,hideCursor
โ this can be easily set via CSSmediaType
,mediaControls
โ you should instead pass an entire media element in themedia
option. Example.normalize
โ peaks are normalized to -1..1 by defaultpartialRender
โ done by defaultpixelRatio
โwindow.devicePixelRatio
is used by defaultrenderer
โ there's just one renderer for now, so no need for this optionresponsive
โ responsiveness is enabled by defaultscrollParent
โ the container will scroll ifminPxPerSec
is set to a higher valueskipLength
โ there's noskipForward
andskipBackward
methods anymoresplitChannelsOptions
โ you should now usesplitChannels
to pass the channel options. Passheight: 0
to hide a channel. See this example.xhr
,drawingContextAttributes
,maxCanvasWidth
,forceDecode
โ removed to reduce code complexity
Removed methods
getFilters
,setFilter
โ as there's no Web Audio "backend"drawBuffer
โ to redraw the waveform, usesetOptions
instead and pass new rendering optionscancelAjax
โ ajax is replaced byfetch
loadBlob
โ useURL.createObjectURL()
to convert a blob to a URL and callload(url)
insteadskipForward
,skipBackward
,setPlayEnd
โ can be implemented usingsetTime(time)
exportPCM
is renamed togetDecodedData
and doesn't take any paramstoggleMute
is now calledsetMuted(true | false)
setHeight
,setWaveColor
,setCursorColor
, etc. โ usesetOptions
with the corresponding params instead. E.g.,wavesurfer.setOptions({ height: 300, waveColor: '#abc' })
See the complete documentation of the new API.
Questions
Have a question about integrating wavesurfer.js on your website? Feel free to ask in our Discussions forum.
FAQ
- Q: Does wavesurfer support large files?
- A: Since wavesurfer decodes audio entirely in the browser, large files may fail to decode due to memory constrains. We recommend using pre-decoded peaks for large files (see this example). You can use a tool like bbc/audiowaveform to generate peaks.
Development
To get started with development, follow these steps:
- Install dev dependencies:
yarn
- Start the TypeScript compiler in watch mode and launch an HTTP server:
yarn start
This command will open http://localhost:9090 in your browser with live reload, allowing you to see the changes as you develop.
Tests
The tests are written in the Cypress framework. They are a mix of e2e and visual regression tests. To run the test suite locally:
yarn cypress
Feedback
We appreciate your feedback and contributions! Join the conversation and share your thoughts here: #2789
If you encounter any issues or have suggestions for improvements, please don't hesitate to open an issue or submit a pull request on the GitHub repository.
We hope you enjoy using wavesurfer.ts and look forward to hearing about your experiences with the library!